Writing about my shell init display. Why?
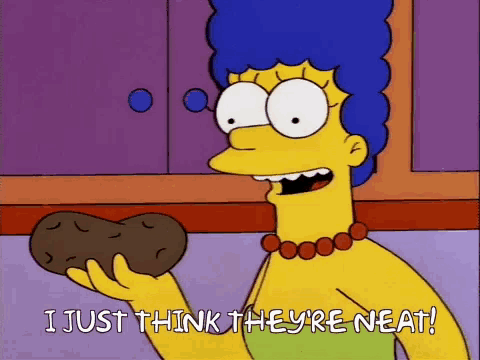
Marge thinks they are neat
What?
So, when my shell starts up, it loads a bunch of modules, draws some fun stuff, and shows an in-line image based on the OS (using iTerm2’s image display protocol).
How?
First part, the modules. When my shell starts, it loads up all the files in
$HOME/.zsh/modules.d/
. This is just a simple loop through the directory–a
normal unix-y list of files with a number at the front to indicate load order
(e.g. 00-loadme-first.zsh
or 99-unimportant.zsh
). It then iterates over
their initialisation functions. This is done in two phases, since some of the
modules create a global array or register helper functions that other modules
may depend upon (notably the error handling putting info into the prompt line).
# Load modules from the dynamic module directory.
if [[ -d "$MODDIR" ]]; then
for mod in ${MODDIR}/*.zsh; do
source $mod
done
fi
...
print -P "${CL_NORM_ON}Initializing modules...${CL_NORM_OFF}"
for mod_name mod_init in ${_aimee_zsh_modules}; do
print " $mod_name"
eval $mod_init
done
print
These modules are created using my own module format/convention. They call a
function module_add
with a title and reference to an init function. For
example, the module 80-iterm.zsh
calls module_add "iTerm2" _aimee_iterm_init
. Note that not all modules use this, notably the text image
itself, which runs immediately upon load (so the print during initalisation is
put inline along the image).
The text image (in 00-banner.zsh
link) is drawn using a
combination of zsh colour codes and terminal escape sequences to move the
cursor around. The cursor is moved back up to the top-left of where this
sequence started (just below the ruler). This is so that the subsequently
loaded modules will announce themselves along side the image.
IFS="" read -r -d '' TEXT << '__EOF'
%F{magenta}0---------1---------2---------3---------4---------5---------6---------7--------7%f
%F{magenta}01234567890123456789012345678901234567890123456789012345678901234567890123456789%f
DRAWINGS GO HERE
%f%b
\033[16A
__EOF
print -P "${TEXT}"
The actual image portion (in 98-term-ident.zsh
link) is done
using iTerm2’s image support. The script tries to be smart and show the
stylised ascii OS name along side a blank space when running within a terminal
that does not support real images (e.g. Terminal.app or tmux). This is the
essential bit of code, where the text is written, the curosr is moved back up
to the top, and then either the PNG or a few blank lines are rendered.
case "${SYSNAME}" in
Darwin)
# src file: "distro-darwin.png"
IMGDATA="BASE64-ENCODED-PNG-FILE-GOES-HERE"
IFS="" read -r -d '' TEXT << '__EOF'
____ _
/ __ \____ _______ __(_)___
/ / / / __ `/ ___/ | /| / / / __ \
/ /_/ / /_/ / / | |/ |/ / / / / /
/_____/\__,_/_/ |__/|__/_/_/ /_/
\033[7A
__EOF
;;
...
esac
if [[ -n "${IMGDATA}" ]]; then
echo
echo "${TEXT}"
if [[ "${LC_TERMINAL:-}" == "iTerm2" && -z $(__iterm2_is_tmux) ]]; then
__iterm2_print_image "" 1 "${IMGDATA}" 0 "" "5" "1" ""
else
echo; echo; echo; echo; echo
fi
echo
fi
Show me!
What’s the result of all this? Here is what launching a new shell instance looks like on two different systems: my macOS laptop, and, a Linode I have configured a Tailscale exit node.
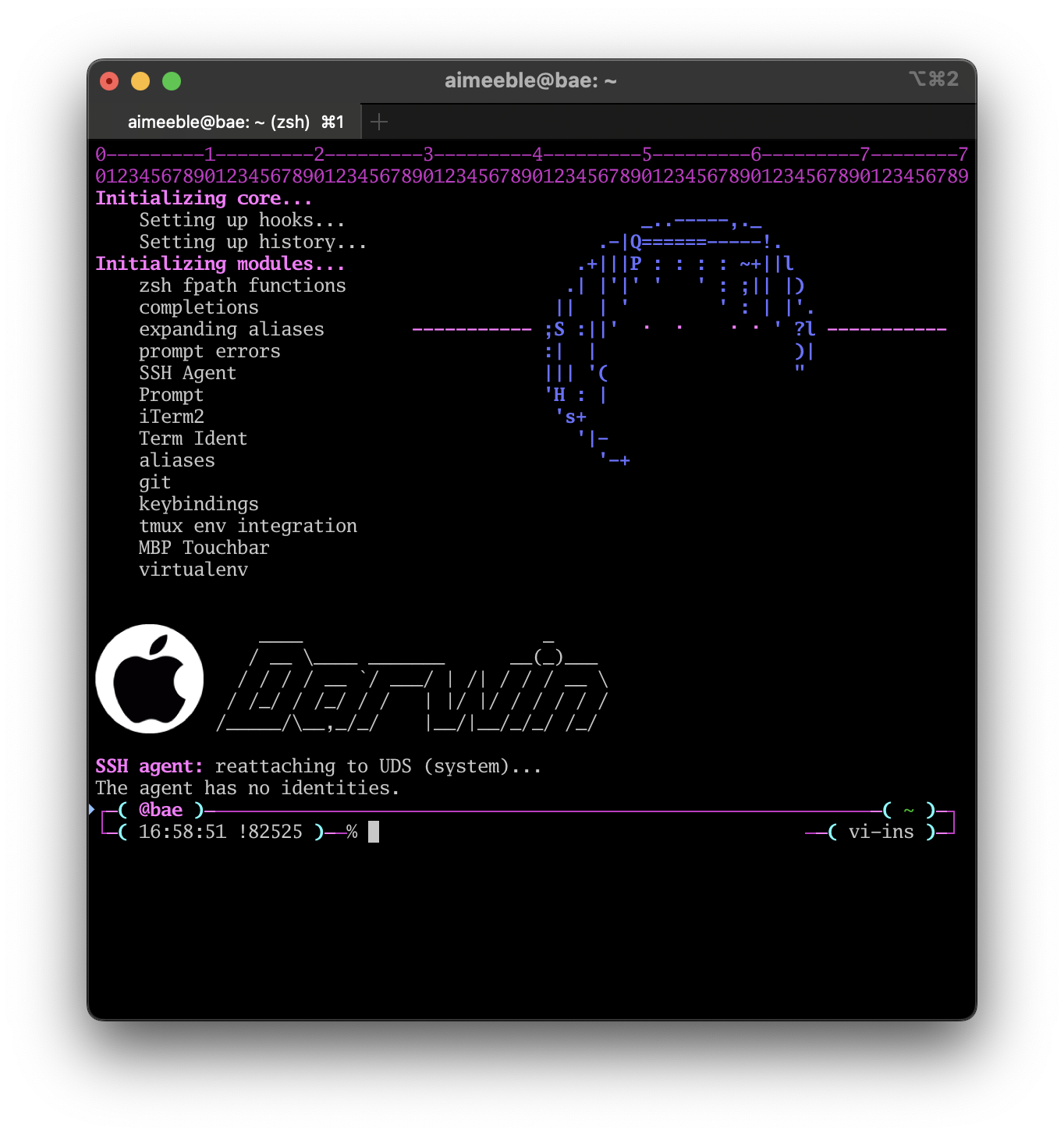
Darwin
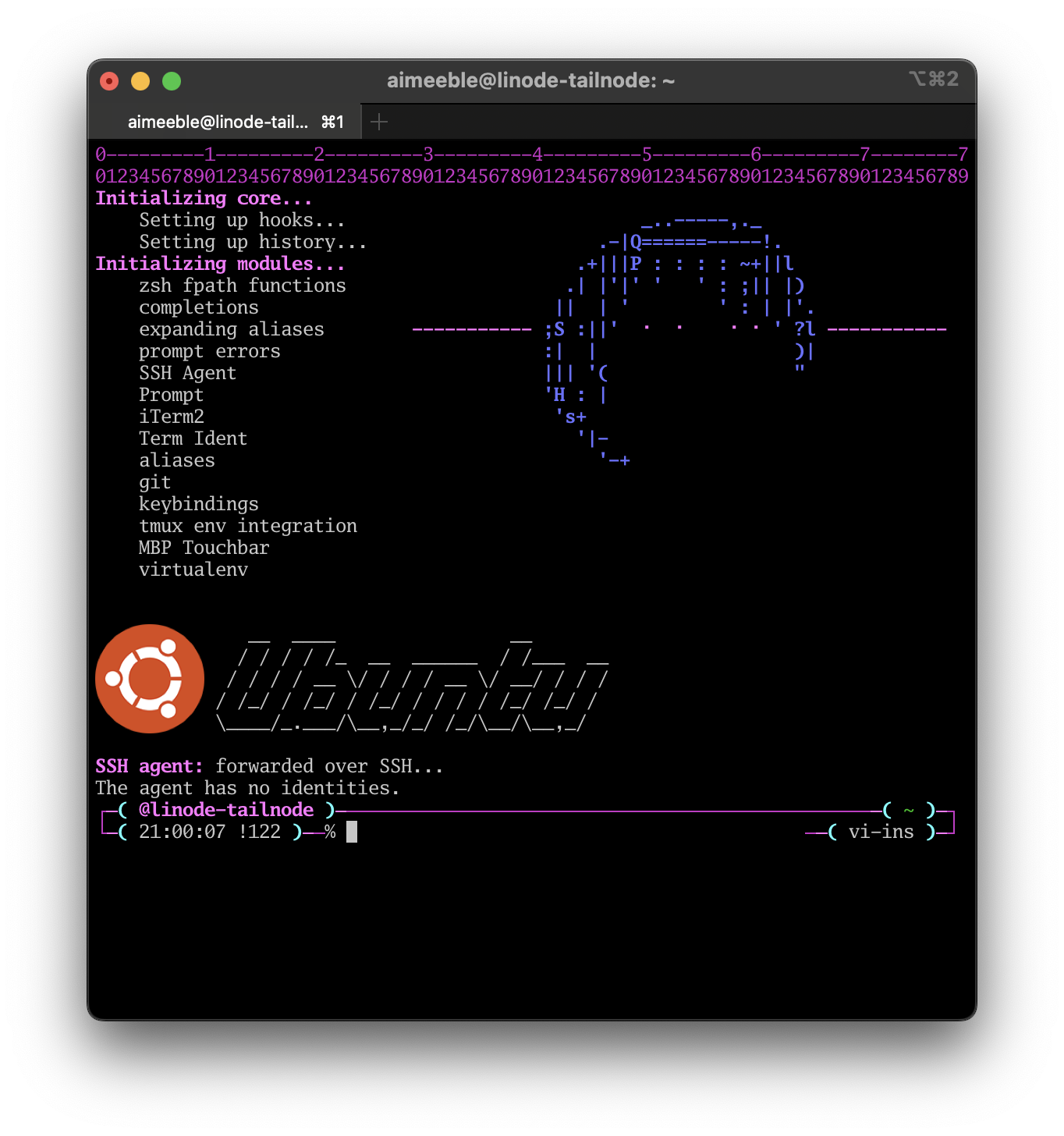
Ubuntu
Update 2025-01-10: I’ve added a bunch of different little doodles that appear next to the loading modules. One is choosen at random when zsh launches/loads my dotfiles.
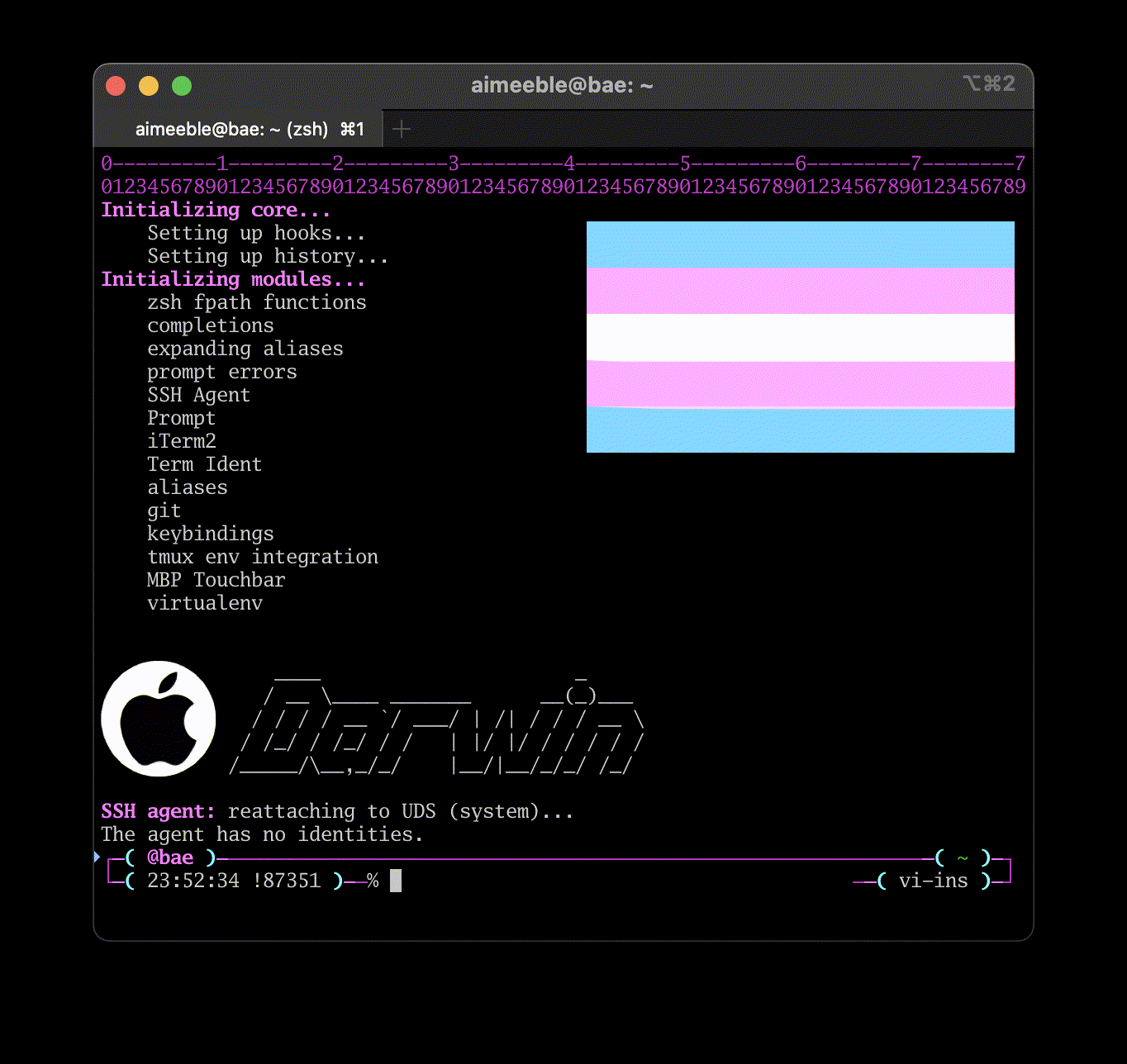