Creating the game framework
As I mentioned in the previous #devlog, I’m starting things out with a Pac-Man clone and I’m building it with Fyrox. Fyrox comes with Fyroxed, an editor for game scenes.
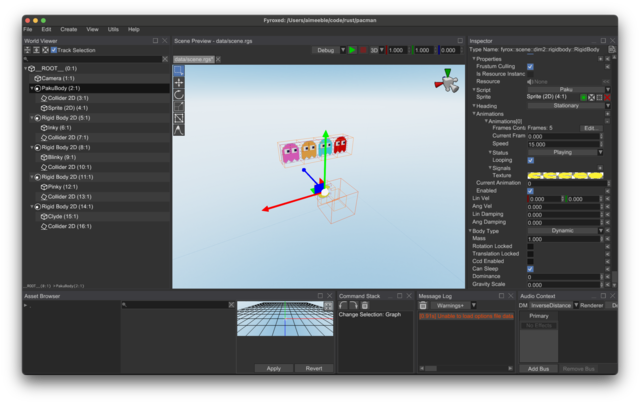
Fyrox Editor
For this simple game, the scene only has the moving elements (player and ghosts). The playfield itself is constructed programmatically. Each tile is a quad with texture (rendering 2D rectangles is very optimised in Fyrox, so use all the rectangles).
I’ve still got to decide how I’m going to represent the playfield internally, which will be used for both derriving the visuals and feeding into the ghosts' pathfinding algorithms. Right now, it’s literally a 2D-array of ints representing the tile type
Input
Handling the user input is pretty straight forward, since the player character can only move on the four cardinal directions, and once moving, just keeps moving until there is a collision with a wall. This means I only need to track the most recently requested direction, and then when the game updates its state, just move the player along in that direction. Easy peasy.
fn on_os_event(
&mut self,
event: &Event<()>,
_context: &mut ScriptContext,
) {
if let Event::WindowEvent { event, .. } = event {
if let WindowEvent::KeyboardInput { event: input, .. } = event {
if let PhysicalKey::Code(code) = input.physical_key {
match code {
KeyCode::KeyW => self.heading = Heading::North,
KeyCode::KeyA => self.heading = Heading::West,
KeyCode::KeyS => self.heading = Heading::South,
KeyCode::KeyD => self.heading = Heading::East,
_ => (),
}
}
}
}
}
User input handling
What’s it look like now?
Right now, I haven’t done much art, so the textures are not set – I justed set each tile type to a distinct colour (so I could at least tell if I mapped things out right; can you find where I did not set things correctly?). Also, there is no collision detection yet (look at yellow-square go! walls cannot stop it!)
Next steps will be:
- Get some minimal textures. Probably for the player character first, since it’s got animations that will require iterating over a sprite sheet.
- Add collision detection between characters and the playfield.
- Get the ghosts moving.
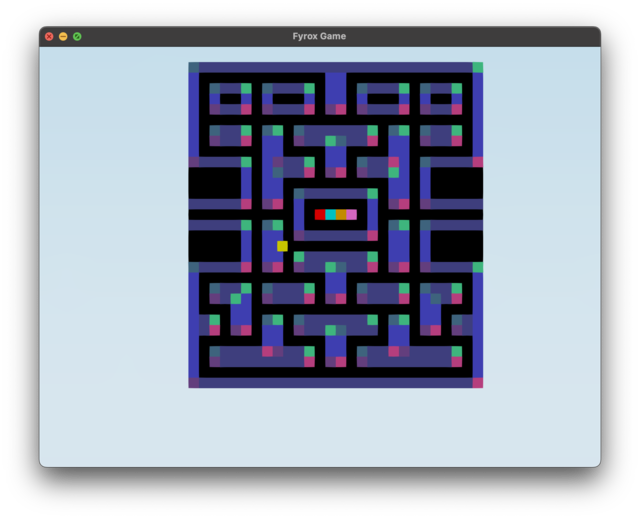